What is Web3.js? Exploring the Basics of Web3.js
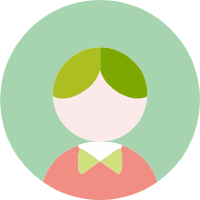
Web3.js is a JavaScript library that enables developers to build decentralized applications (DApps) and interact with blockchain networks. It is a core component of the Web3 ecosystem, which aims to create a transparent, secure, and decentralized web. In this article, we will explore the basics of Web3.js, its purpose, and how it can be used to develop Web3 applications.
Web3.js Overview
Web3.js is a powerful JavaScript library that provides a set of API's to enable communication with blockchain networks such as Ethereum, Polygon, and Binance Smart Chain. It provides a simple and convenient way to interact with the blockchain, making it easier for developers to build DApps and smart contracts.
Web3.js's primary purpose is to facilitate communication between the user interface (UI) and the back-end smart contracts. It allows developers to create user-friendly interfaces without having to worry about the complex logic of the smart contract. By using Web3.js, developers can easily build and deploy smart contracts on a blockchain network, as well as interact with them through web applications.
Web3.js Features
1. Token abstraction: Web3.js provides a standard interface for working with tokens, allowing developers to easily manage and transmit tokens across different blockchains.
2. Transaction processing: Web3.js allows developers to process transactions sent from the UI, such as creating new accounts, sending tokens, and calling smart contract functions.
3. Events: Web3.js enables developers to listen for events triggered by smart contracts, such as token transfers or contract creation.
4. Account management: Web3.js provides a convenient way to manage multiple accounts and their related private keys.
5. Contract management: Web3.js allows developers to create, deploy, and interact with smart contracts on a blockchain network.
6. Interface translation: Web3.js provides a layer of abstraction that translates web API's into the correct blockchains' interface, allowing developers to build web applications without having to worry about the intricacies of blockchain technology.
Building Web3 Applications with Web3.js
To build a Web3 application, the first step is to install Web3.js on the development environment. Once installed, developers can start creating Web3 applications by following these steps:
1. Install Web3.js: Install Web3.js using npm or yarn by running the following command in the project folder:
```
npm install web3
```
Or
```
yarn add web3
```
2. Set up a web3 provider: Web3.js requires a web3 provider to connect to a blockchain network. The most common provider is MetaMask, which can be added to the project by integrating it with the browser extension.
3. Initialize web3: Once the provider is set up, the `web3.js` library can be used to initialize a new web3 client by running the following code:
```javascript
const web3 = new web3.js('https://your-provider-url');
```
4. Deploy smart contracts: Developers can use Web3.js to deploy smart contracts to a blockchain network by providing the contract's bytecode and ABI (Application Binary Interface).
```javascript
const contract = await web3.js.deploy({
// contract code and abi
});
```
5. Interact with smart contracts: Once the smart contract is deployed, developers can use Web3.js to call functions, get events, and manage tokens using the web3 client.
```javascript
// invoke a smart contract function
const result = await web3.js.contract({}).method('functionName').call();
// get events from a smart contract
const event = await web3.js.contract({}).method('eventName').getEventData();
// transfer tokens
await web3.js.contract({}).method('transfer').send({ from: '0xyourAddress', to: '0xrecipientAddress', value: '100000000000000000' });
```
Web3.js is a powerful tool that enables developers to build decentralized applications and interact with blockchain networks. By understanding its core features and applications, developers can create robust and secure Web3 applications that revolutionize the way we access and use digital assets. As the Web3 ecosystem continues to grow, Web3.js will undoubtedly play an essential role in shaping the future of the web.