Python Web Server CGI Example: A Comprehensive Guide to CGI Programming in Python
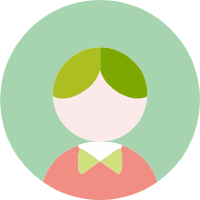
Python is a highly versatile and powerful programming language that is increasingly being used for web development. One of the most common ways to create web applications using Python is through the use of the Common Gateway Interface (CGI). CGIs allow web servers and web applications to communicate with each other, allowing for the exchange of data and the generation of HTML pages. In this article, we will explore a simple example of using Python to create a CGI application, providing a comprehensive guide to CGI programming in Python.
1. Setting up the environment
Before we can begin writing our Python code, we need to set up the environment for our CGI application. We will be using Python's HTTP package to handle the communication with the web server. To do this, we first need to install the HTTP package using pip.
```
pip install http
```
2. Creating the Python script
Now, we will create a simple Python script that will handle the CGI requests. Create a file called `cgi_example.py` and add the following code:
```python
import http
def handle_request(request):
# Replace this with your own logic to process the CGI request
response = "Hello, World!"
return response
if __name__ == "__main__":
server = http.Server(("0.0.0.0", 8000))
server.handle_request = handle_request
server.run_forever()
```
3. Configuring the web server
To have our Python script handled by the web server, we need to configure our web server to use it. In this example, we will use Python's `http.server` module to set up a simple web server.
Create a file called `settings.py` and add the following code:
```python
from http.server import BaseHTTPRequestHandler
class MyHandler(BaseHTTPRequestHandler):
def get_response(self):
return "Hello, World!"
server_address = ('', 8000)
```
4. Running the web server
Now, we can run our web server by executing the following command:
```
python3 cgi_example.py
```
5. Testing the CGI application
To test our CGI application, open your web browser and navigate to `http://localhost:8000/`. You should see the response "Hello, World!" displayed on the screen.
6. Advanced features
Now that we have a simple CGI application working, let's explore some advanced features. We can use Python's `os` and `sys` libraries to access the current working directory and the environment variables, respectively. We can also use the `requests` library to make HTTP requests to other websites.
7. Conclusion
In this article, we have explored a simple example of using Python to create a CGI application. We have seen how to set up the environment, create the Python script, configure the web server, run the web server, test the CGI application, and explore advanced features. By following this comprehensive guide, you should now have a solid understanding of how to use Python for CGI programming. Feel free to explore other Python libraries and frameworks for web development, such as Django, Flask, and FastAPI, to build more sophisticated web applications.